Concurrent Collections
May 5, 2020

Remember the idea of thread safe, having to do extra work to make sure that two different threads don’t run the same code at the same time if that code access shared state. Well, concurrent collections take of that for you to a significant degree. It’s not perfect, but it’ll go a long way to writing code where one thread safely talks to another thread through shared state, shared collections, and so these collections are designed to be used concurrently, to be used for multiple threads at the same time.
Lock and Lock-free types
In the .NETFramework, we have some types that are done with locking, and some types, some of the concurrent collections, done completely lock-free. Now when I say lock-free, I don’t mean that there is absolutely no sharing or coordination going on. No, like on the concurrent queue and concurrent stack, they use interlocked operations. There’s an interlock class. The interlock class has a method called increment, decrement, and exchange. So, depending on how you design your algorithm, you may be able to use these methods that are atomic operations to achieve sharing or coordination, or as we say here, interlocked operations, so that multiple threads can access shared state in a way that they’re not going to step on each others toes.
In the concurrent collections namespace, we have a concurrentqueue, works similar to a traditional queue, you add items first in, and when you read items, it’s the first one that went in that’ll be the first one coming out, FIFO. We also have a stack, so first in, last out, and both of these can be used from multiple threads.
we also have the ConcurrentDictionary. Now, the dictionary works like a traditional one, except that we are going to allow multiple threads to add and remove items in this dictionary.
Common pattern
Then there is of course, the common patterns. One of the most common patterns is where we have a producer, one or more threads that’s producing content, data, something that other threads want to consume. And so there is this pattern called producer-consumer, where we have a producer generating data, placing it, say, into a queue or a dictionary, or similar, and then readers or consumers of that content, reading from or retrieving from that shared data structure. There is a built in data type for this, and it is called Blocking Collection.
ConcurrentQueue

ConcurrentDictionary
Using ConcurrentDictionary
to count number of each word in a document.
By using AddOrUpdate
we could increment current value of each word count during processing the document.
Producer Consumer Pattern with BlockingCollection
using BlockingCollection
lets us to have a certain capacity of items in collection. by using Add
it will wait until collection will has free capacity and by using Take
the process will wait until new item would be available
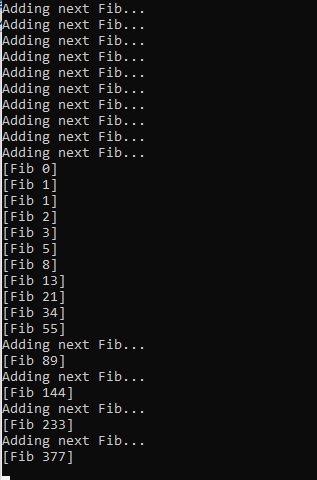