Multithreading
April 26, 2020
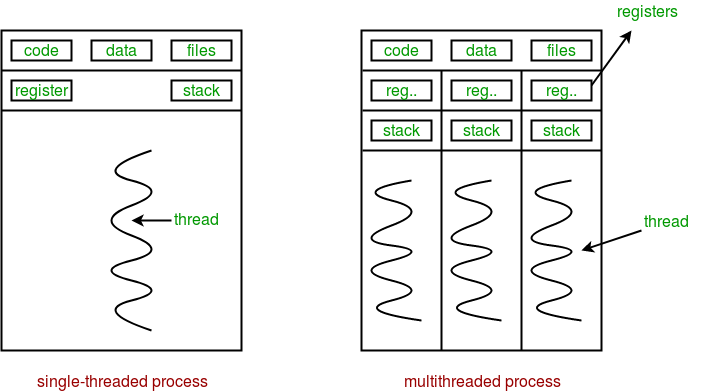
Threads vs. Processes
Processes run in parallel on a computer. In a very similar fashion, threads run in parallel except just within a single process. One thing to keep in mind is that processes are fully isolated from each other, whereas the thread have a limited degree of isolation. Thread share heap memory with other thread running in same application (process), even though they have local variables, which actually are part of the local memory that can not be accessed by other threads. However with shared memory, shared resources could simply be fetched, for example, by a background thread while, let’s say, the foreground thread or the main thread can actually display the data as it arrives.
Shared resources
Watch threads in visual studio
You can see threads and active thread in visual studio in debug mode. Run the application, set a break point. Now go to menu Debug > Windows > Threads to open threads windows:
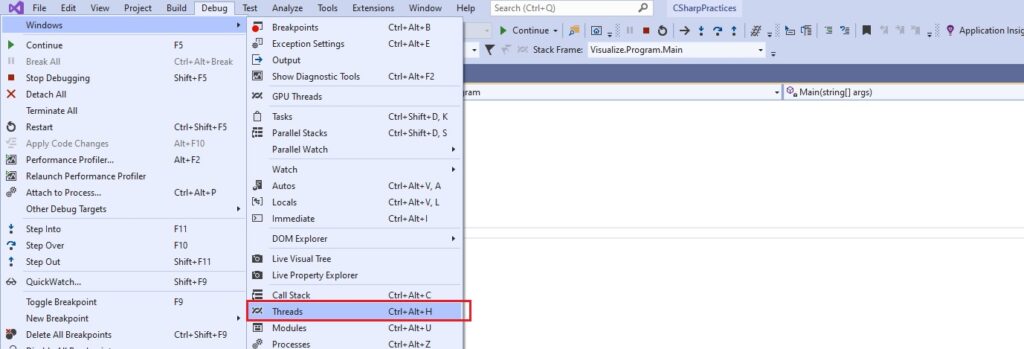

Thread Pool
Every single thread requires a certain overhead and it’s about a few hundred milliseconds. This is usually the time spent in creating a flash local variable stack and spawning off the thread. Every individual thread also consumes roughly around one megabyte of memory.
One of the major benefits of something like thread pool is to reduce the performance penalty by sharing and recycling threads.
One thing to keep in mind is that a thread pool only creates background threads.
Now what’s a background thread?
A background thread is almost identical to foreground thread by default. The only have one exception. That they do not keep the managed execution environment running. So if the main thread dies, the background threads are going to die. So the application crashes and background threads will terminate abruptly. in order to create a background thread, we simply create a new thread and then we use the is background property and set it to true.
The thread pool is responsible to keep and upper count on the total number of worker threads can run simultaneously. So let’s say the count is five, it will not allow more than five threads run in parallel.
So what’s happen once the limit is achieved? When the limit is achieved, all the jobs queue up and start only when another job finishes. Now how do you find out if you are currently executing on a pool thread? we use Thread.CurrentThread.IsThreadPoolThread
property.
What are the different ways to enter a thread pool?
– Task parallel library
– Asynchronous delegates
– Create a background worker
– Call ThreadPool.QueueUserWorkItem
Wait to thread ended
In some scenarios we need to wait to a thread finished and then do something like print a statement. one way is to use thread.join()
Exception handling
Exception handling is per thread.