Synchronization
April 27, 2020
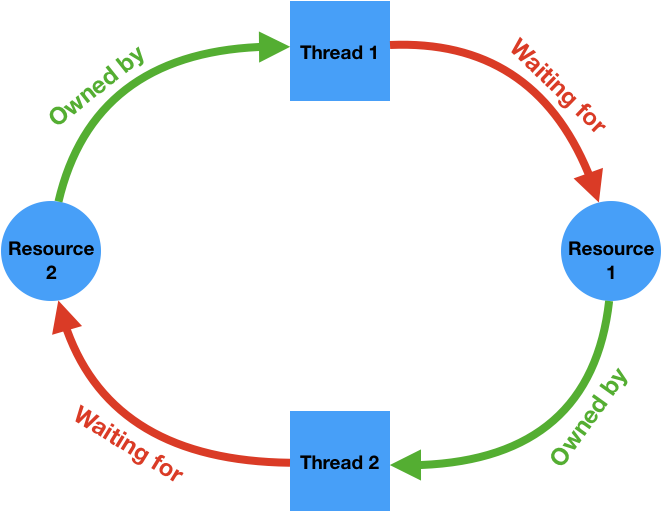
Exclusive Locks
lock(someObject){}
Allow only one thread to access a certain section of code. unless and until the thread has not finished executing that code, no other thread will be able to enter.
Alternative is to use Monitor.Enter
/Monitor.Exit
it’s only syntactical difference. behind the scenes, they have the same exact implementation.
Two types of exclusive locks
- Lock
- Mutex
If we give a name to a mutex , that mutex would be accessible computer-wide: var mutex = new Mutex(false, "mutex name");
for access protected code by mutex we have to ask if it’s available by : mutex.WaitOne();
there is also another option which allow us to wait for certain amount of time and if the mutex was not released in that amount of time the thread will not wait anymore: mutex.WaitOne(certainAmountOfMilliSeconds);
Nonexclusive Locks
- Semaphore
- SemaphoreSlim
- Reader/Writer locks
Allow multiple threads to access resources.
Nonexclusive locks add a limit of the number of threads accessing a particular resourceSemaphore
and SemaphoreSlim
ensure that not more than a specified number of concurrent threads can access a particular resource.
SemaphoreSlim is lighter version of Semaphore (with less overhead to create one: about 200 nanoseconds)
Signaling Constructs
Signaling constructs allow a thread to pause until they receive a notification from another thread. So if thread A wants to do something only after receiving a notification from thread B, it could actually wait, and the moment B is done, it could call a set method and let thread A know that now it can continue execution. So it’s very helpful when you want thread A to execute certain lines of code and then wait for some time before it executes the following line of codes.
Two commonly used signaling devices:
- Event wait handles
- Monitor Wait/Pulse
The .NET Framework 4 also introduced:
- Countdown Event
- Barrier classes
Nonblocking Synchronization
- Thread.MemoryBarrier
- Thread.VolatileRead
- Thread.VolatileWrite
- vloatile keyword
- interlocked class