Thread Signaling
April 28, 2020

.NET Framework built-in static methods are thread safe.
When creating static methods, it is a best practice to make them thread safe.
Thread Affinity
Thread that instantiate an object is the only thread that is allowed to access its members. (Like WPF UI thread)
One of the advantages of having thread affinity is that we may not need to use a lock to use instantiated object, since no other thread can access them.
What is Signaling?
Synchronizes shared resources among threads.
Is used to notify another thread that it can now access a resource that was being used by the current thread.
3 kinds of EventWaitHandles for signaling
- AutoResetEvent
- ManualResetEvent
- CountdownEvent
AutoResetEvent
AutoResetEvent is used when thread needs exclusive access to a resource, and they communicate their intent by signalling. It’s very similar to a ManualResetEvent except it reset itself automatically. An AutoResetEvent is like a baffle gate. Only one person can pass through at any given point of time, we can only have one thread access a resource. It automatically closes right after someone step through, that’s why it’s called AutoResetEvent.
– A thread waits for a signal by calling WaitOne
.
– Calling Set
signals release of waiting thread.
– If multiple threads call WaitOne
, a queue is formed.
– If Set
is called when no thread is waiting, the handle stays open indefinitely.
– Calling Set
only release one thread at a time, regardless of how many times it is called.
– Can create an AutoResetEvent with an initial state of signaling by passing true
, if you pass in false
, the AutoResetEvent will be in a non-signal state.
– Simply creating by false
means no thread can enter until some where Set
called.
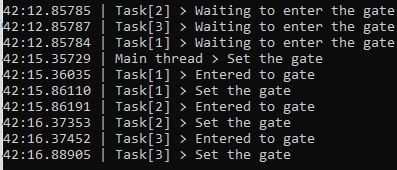
Assume we change the first line of initialization with:static System.Threading.AutoResetEvent eventWaitHandle = new System.Threading.AutoResetEvent(true);
Then the result would like:
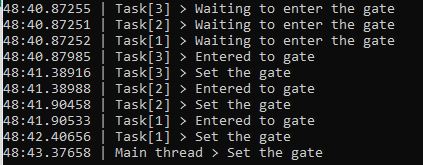
Two way signaling
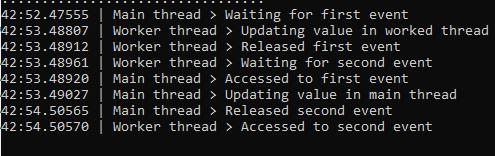
ManualResetEvent
So if you were to take the analogy of the baffle gate where only one could make it, ManualResetEvent is like a different gate where once the gate is open, any amount of threads can go past the gate at the same time. And of course we have to Set
and Reset
the gate manually. In case of AutoResetEvent once we call Set
, we do not have to call Reset
.
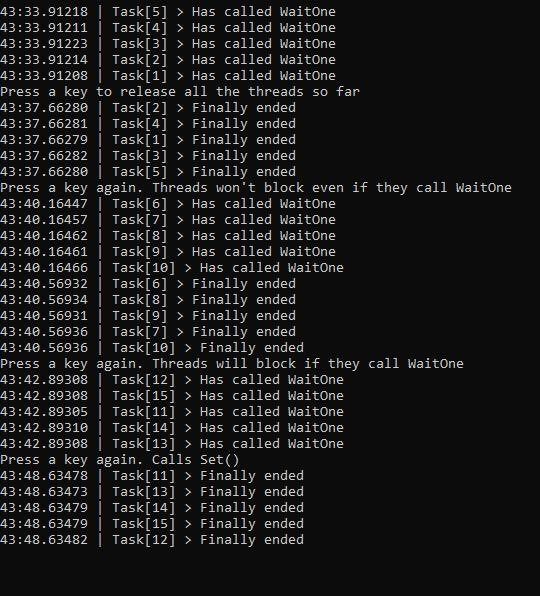
CountdownEvent
In instantiate this event we specify number of threads for countdown, this event has a method named Signal
, once all number of threads called Signal
another thread waits for this event will be released.
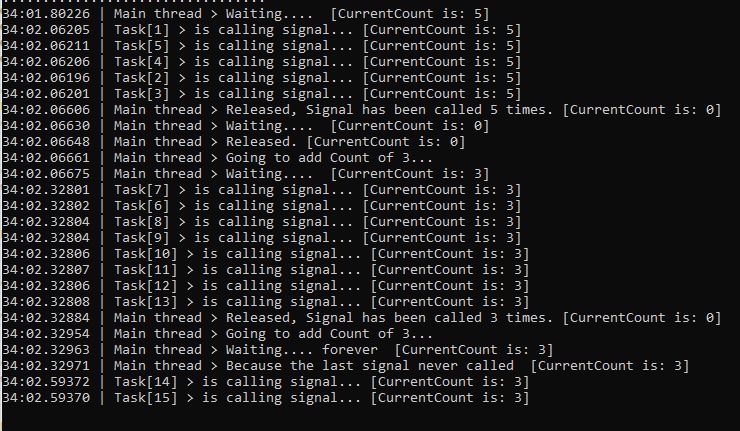