Design Patterns Part 1
May 25, 2020
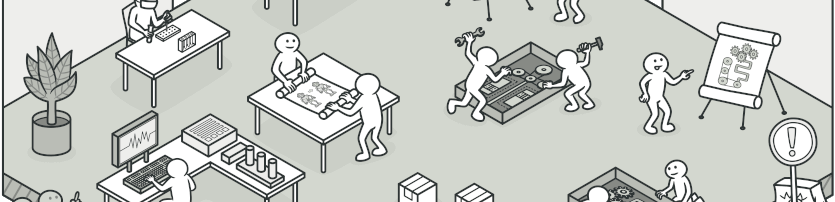
There are three categories in design patterns:
- Creational
- Structural
- Behavioral.
Creational patterns provide a way to make objects while hiding the creation logics, rather than instantiating objects directly. In short, they deal with object creation mechanisms.
Structural patterns are all about class and object composition. This design pattern tends to ease the design by identifying a simple way to realize relationships between entities.
Behavioral patterns are specifically concerned with communication between objects, that is, how objects communicate with each other.
Design Pattern Categories
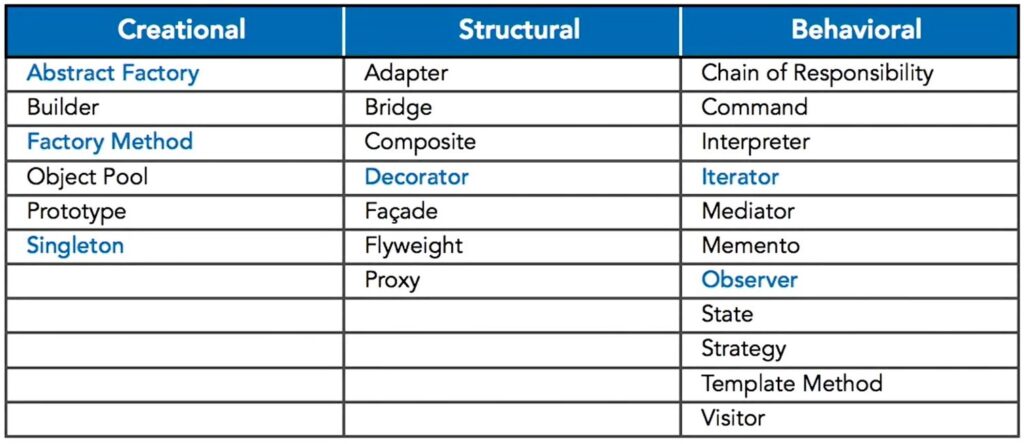
Part 1, 2, 3
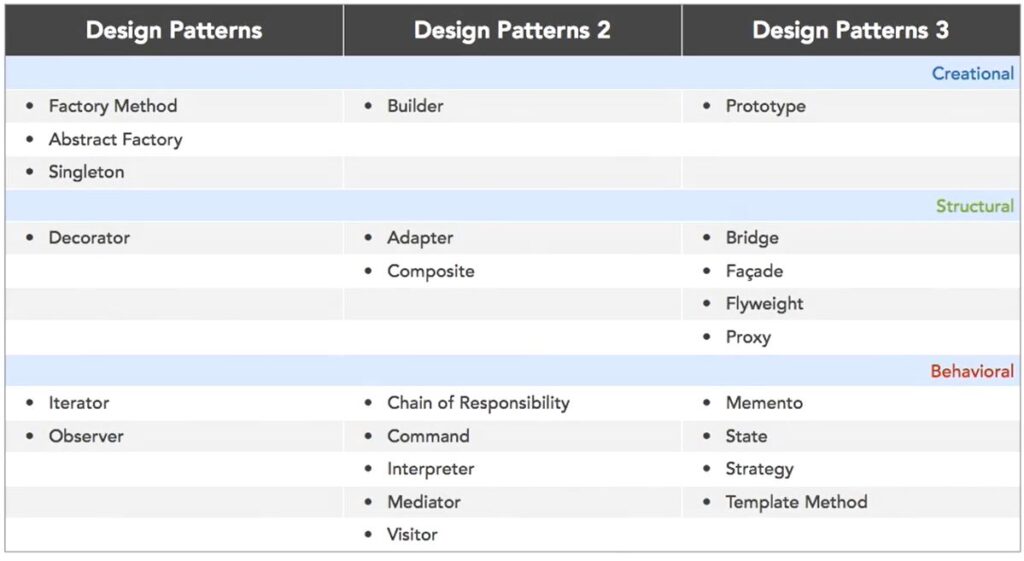
Factory Method
Defining an interface for creating an object but let subclasses decide which class to instantiate. Factory method lets a class defer instantiation to subclasses.
Gang of Four
This pattern is simply about requesting objects that are created behind the scenes and having the correct type of the object returned to you.
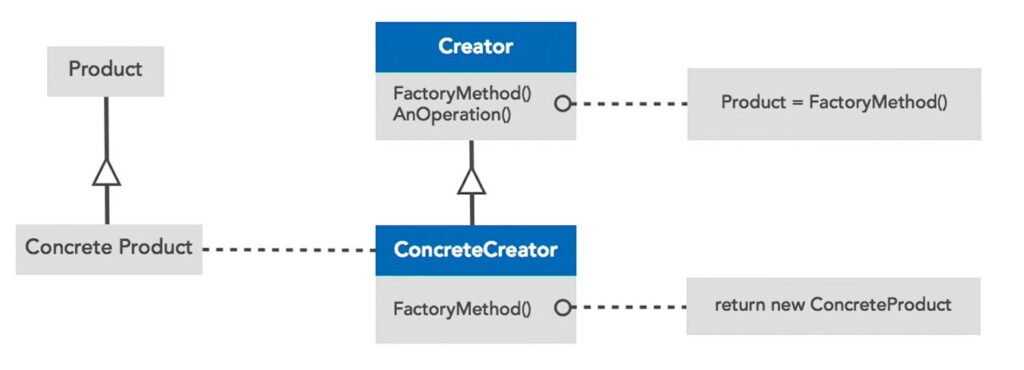
Sample
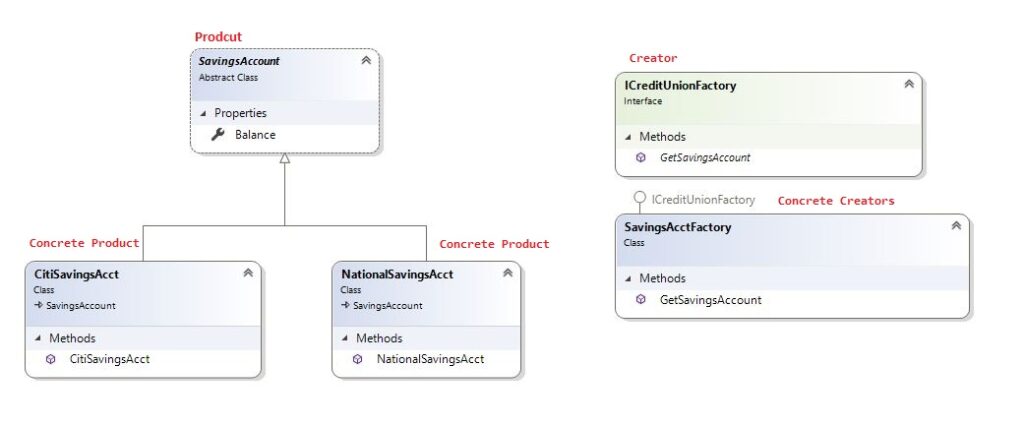
Abstract Factory
Pproviding an interface for creating families of related or dependent objects without specifying their concrete classes.
Gang of Four
you can think of the abstract factory pattern as a factory for creating factories, it’s like the factory method pattern, but everything is encapsulated, it’s a higher level of abstraction using interfaces and abstract classes.
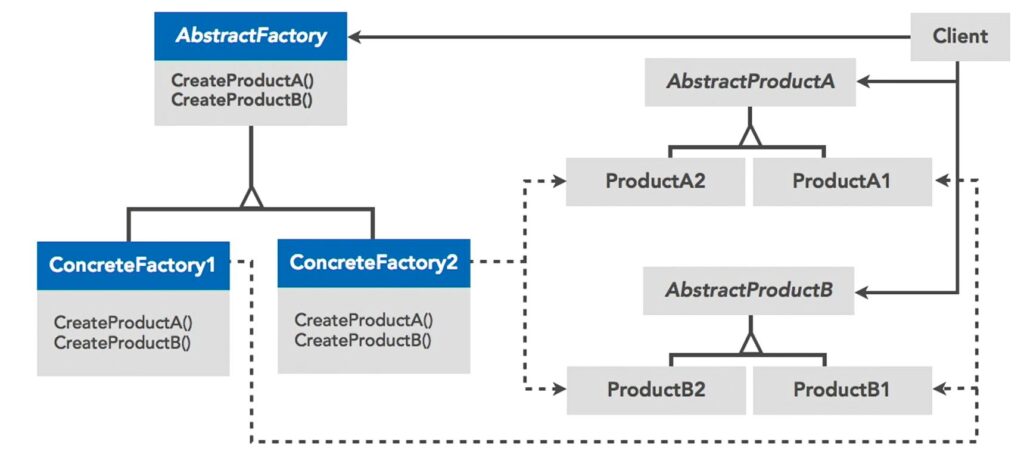
Sample
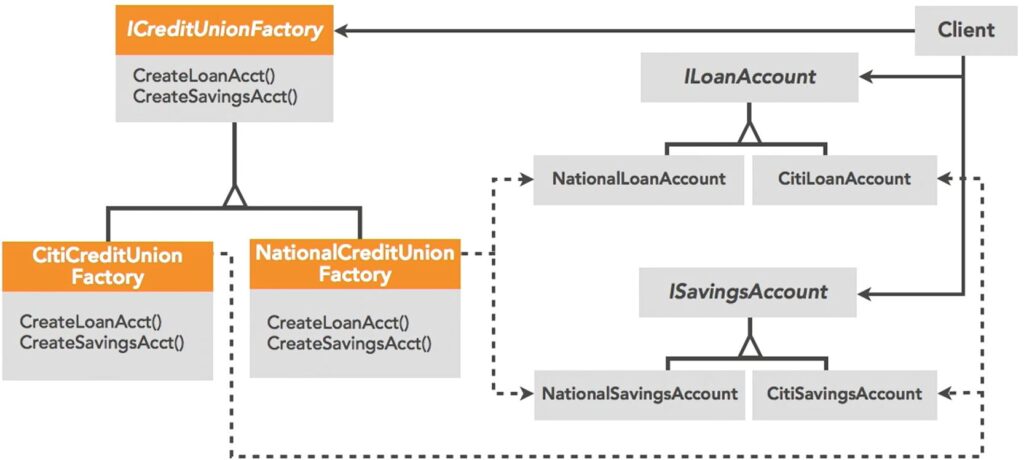
Singleton
Ensuring a class has only one instance, and provides a global point of access to it.
Gang of Four
Singletons are used when you want to eliminate the option of instantiating more than one of the same object. So for example, if you log in online to your insurance company’s website to make a few changes to your policy, there should be only one instance of that policy object during the whole time that you’re logged on making changes.
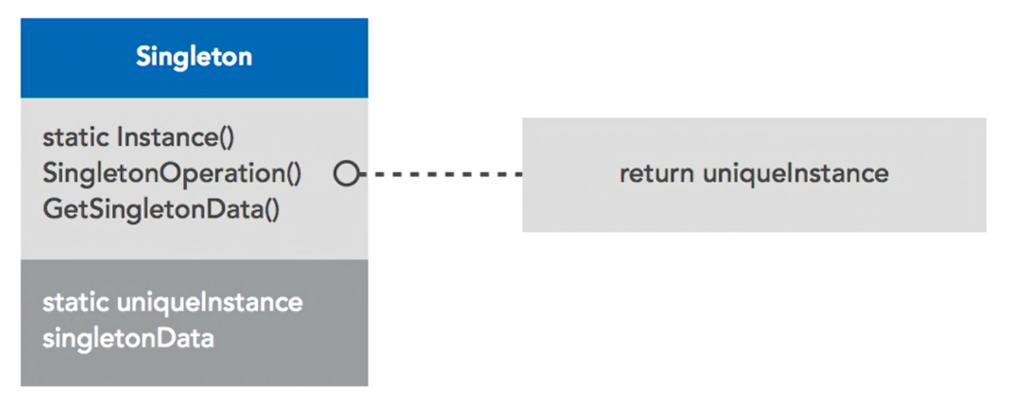
Sample
Decorator
The decorator pattern attaches responsibility to an object dynamically. Decorators provide a flexible alternative to subclassing for extending functionality.
Gang of Four
Decorator pattern is all about using composition and limiting inheritance to simplify object relationships, making them easier to update or manage.
The process boils down to wrapping objects. In fact, sometimes the decorator is called the wrapper pattern.
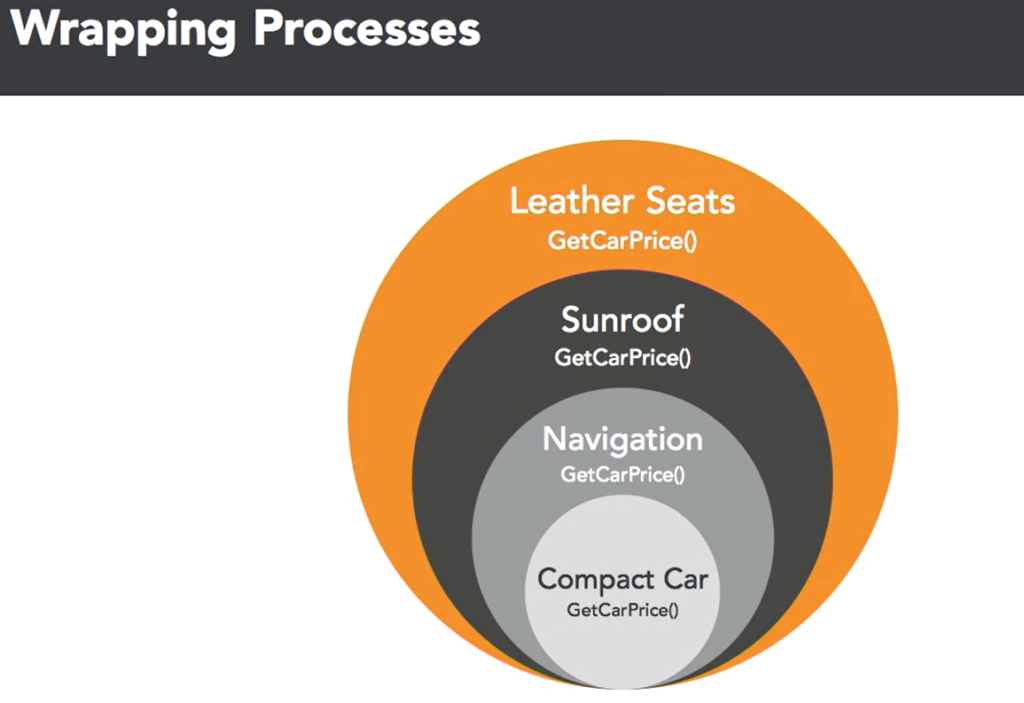
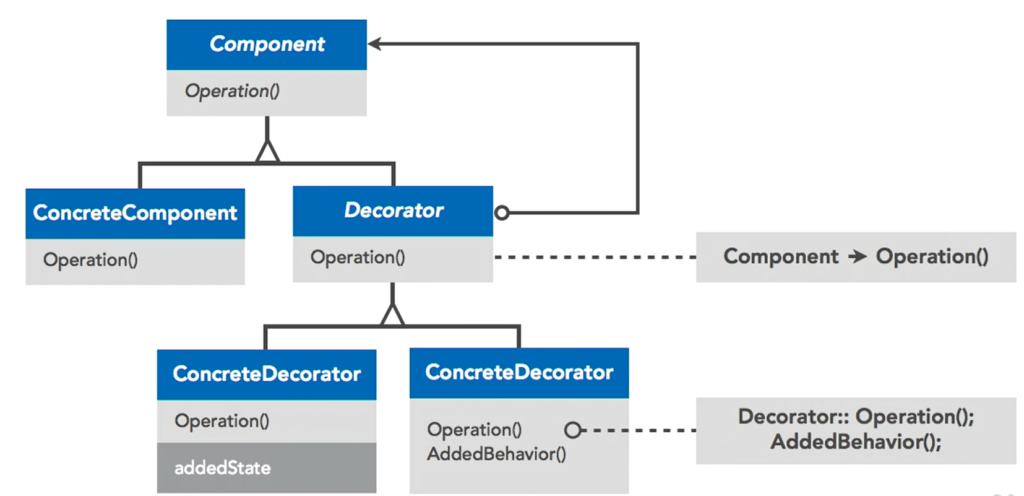

Iterator
The Iterator Pattern is described as providing a way to access the elements of an aggregate object sequentially without exposing its underlying representation.
It’s a way to access and iterate through elements of a collection in the same way despite the type of collection, be it an array, dictionary list, or whatever. Note that some times iterating is often referred to as traversing and the collection can be referred to as a container, or an aggregate.
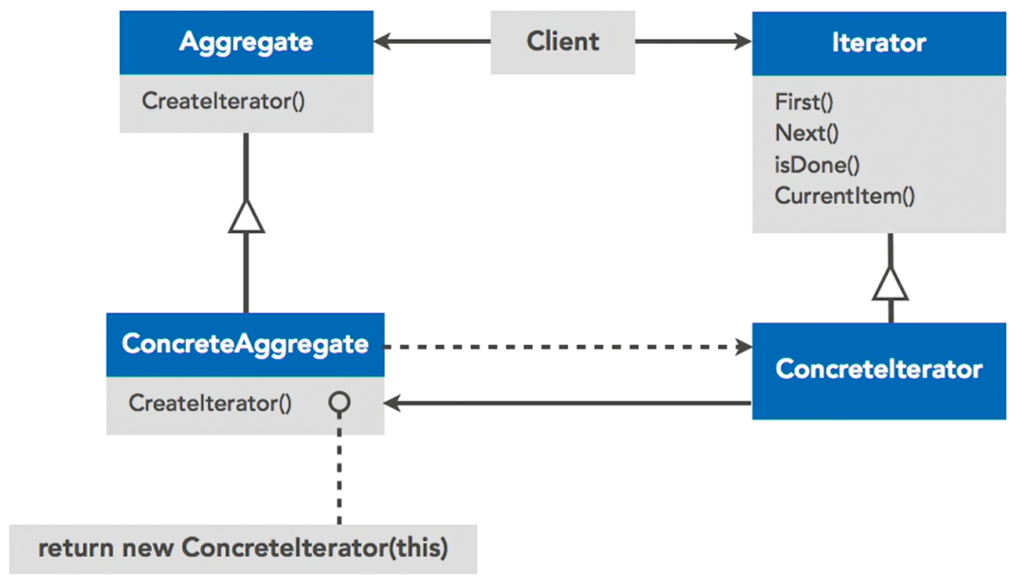
Observer
The observer pattern is described as defining a one-to-many dependency between objects, so that when one object changes state, all its dependents are notified and updated automatically.
The change in one object causes a change or action in another by notifying it.
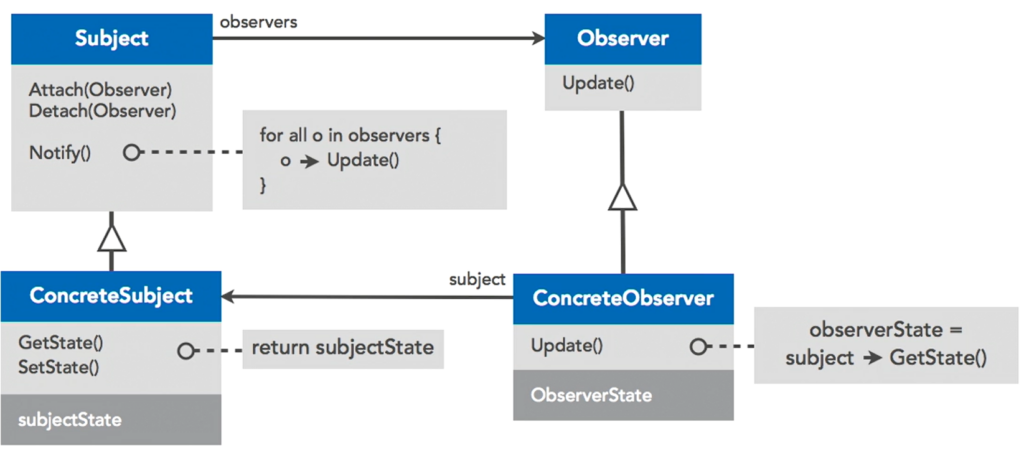
Sample
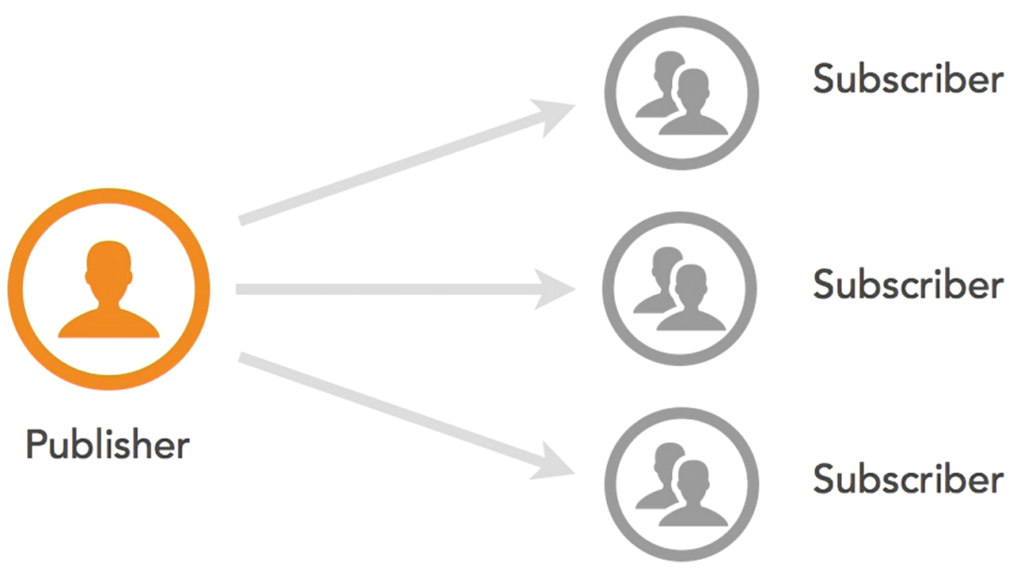
In Windows, there’s event handling which uses the observer pattern. The clicking of a mouse on an image can cause an event to occur, because in the code, there was an object subscribed to a publisher object which notified it to take some action like closing or opening a window.

Repository
A Microsoft MSDN website, it describes a repository pattern as separating business logic from interactions with the underlying data sources. In short, using a repository allows programmers to create an abstraction between an application and its data layer.
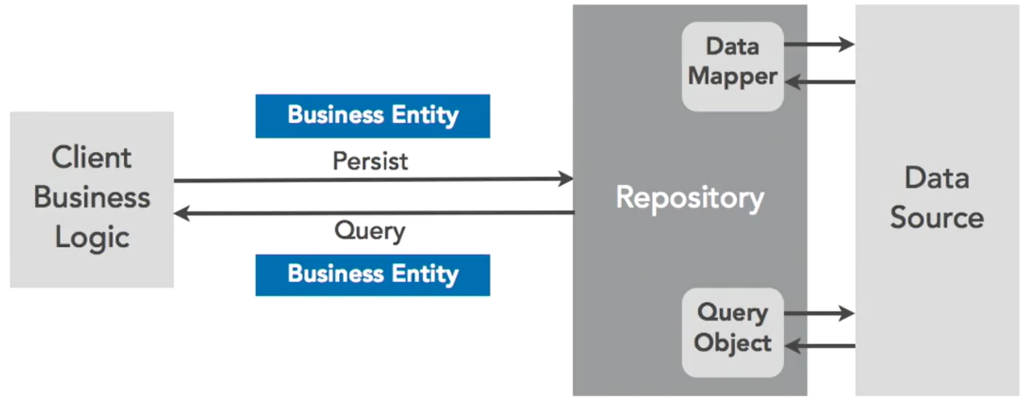
Unit of Work
“Patterns of Enterprise Applications Architecture.”
A list of objects affected by a business transaction which coordinates the writing out of changes and the resolution of concurrency problems.
Basically it allows programmers to update and keep track of data changes in several objects, like multiple repositories, to save to the database in one transaction instead of multiple transactions for every change.
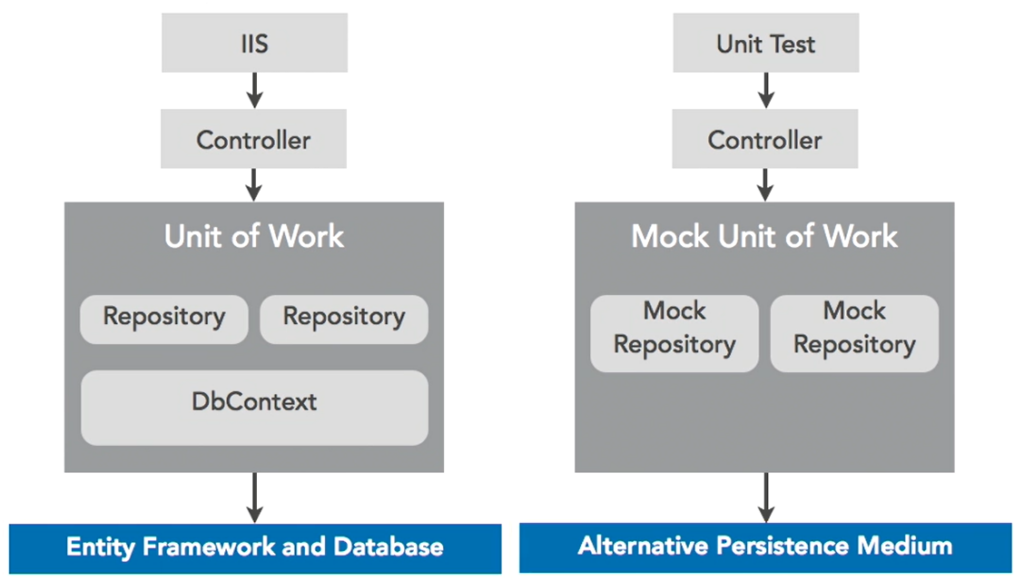