OOP Examples in C#
May 14, 2020
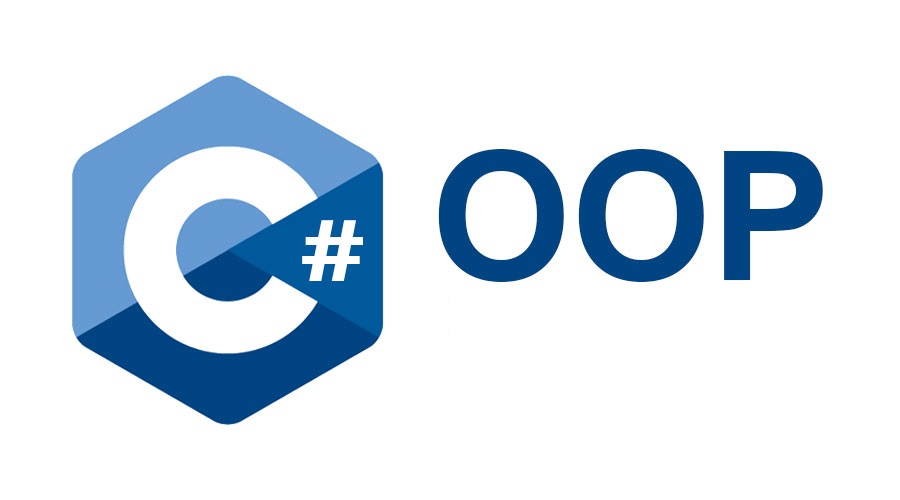
Inheritance or Composition: Two approaches Inheritance Composition IEnumerable IDisposable IEnumerable and Yield Use StreamReader Base Class
Tips & Thoughts
May 14, 2020
Inheritance or Composition: Two approaches Inheritance Composition IEnumerable IDisposable IEnumerable and Yield Use StreamReader Base Class
May 5, 2020
Remember the idea of thread safe, having to do extra work to make sure that two different threads don’t run the same code at the same time if that code access shared state. Well, concurrent collections take of that for you to a significant degree. It’s not perfect, but it’ll go a long way to writing code where one thread safely talks to another thread through shared state, shared collections, and so these collections are designed to be used concurrently, to…
May 1, 2020
Delegates have been available since the start of C# and actually offer us the first and easiest way into asynchronous programming. There is a keyword in C# named delegate which compiler will actually generate an entire class. First assume this simple calling delegate and see the result: The C# compiler based on delegate keyword generate a whole class that will be our type-safe means of having a function pointer. in…
April 29, 2020
Returns Task or Task<TResult> Use Async suffix in methods Overloaded to accept cancelation token
April 29, 2020
PLINQ, or Parallel LINQ when is deduced to automate parallelization. As you might have already guessed that it is parallelization using the same linq queries that we are used to. Except is all we do is say dot as parallel and then that query could run AsParallel query. If we were to execute a certain operation in parallel, we would need to partition the work into tasks, execute those tasks on multiple different threads, and then combine the results. The good…
April 29, 2020
The task parallel library (TPL) is a set of public types and APIs.The vision behind the TPL is for the developers to be able to maximize the performance of code while not having to worry about the nitty gritties of threading. TPL makes us productive by Simplifying the process of adding parallelism and concurrency to applications. Scaling the degree of concurrency dynamically. Handling partitioning of the work . Scheduling threads…
April 28, 2020
.NET Framework built-in static methods are thread safe.When creating static methods, it is a best practice to make them thread safe. Thread Affinity Thread that instantiate an object is the only thread that is allowed to access its members. (Like WPF UI thread)One of the advantages of having thread affinity is that we may not need to use a lock to use instantiated object, since no other thread can access…
April 27, 2020
Exclusive Locks lock(someObject){}Allow only one thread to access a certain section of code. unless and until the thread has not finished executing that code, no other thread will be able to enter.Alternative is to use Monitor.Enter/Monitor.Exit it’s only syntactical difference. behind the scenes, they have the same exact implementation. Two types of exclusive locks Lock Mutex If we give a name to a mutex , that mutex would be accessible…
April 27, 2020
Threads are the most low-level constructs when it comes to multithreading. However work with threads could be very challenging. Let’s say I need a sudden value from worker thread return back to the main thread, the only way is using Join and another variable to retrieve value.. It works, but it can get complicated. So tasks come to our rescue. Task is a higher level abstraction and they’re capable of…
April 26, 2020
Threads vs. Processes Processes run in parallel on a computer. In a very similar fashion, threads run in parallel except just within a single process. One thing to keep in mind is that processes are fully isolated from each other, whereas the thread have a limited degree of isolation. Thread share heap memory with other thread running in same application (process), even though they have local variables, which actually are…